Hello Friends,
Welcome to the CodeLila blog. In the last post we learned about React State and how to use the “useState” hook. If you want to read that post here is the link.
React State- The “useState” Hook – CodeLila
In this new post we are going to learn how we can work with multiple react form input and use component state to deal with user input. We will also look the different way to store the values in component state too. If you are developing an React application and you want to take user input, then this post is all about the same. I am going to help you understand the steps needed for dealing with user input and by the end of blog you will have complete understanding on how we can take multiple form input in React and how we can use State to manage the user input values for same. so, with this introduction to the topic lets get started.
Lets take an example, you are building a brand new React application for a company and you got new requirement. The requirement is , “As a User I want to subscribe to the newsletter”.
You started going through that user story and found that you need to build a input form which can take First Name , Last Name and Email as input and user can click on subscribe button after filling those details.
Lets start building that new form together, for demo , Lets not focus on CSS part and making form beautiful as we want to learn about how to deal with React form input and how to use component state for managing that input.
I have created new component named “NewsLetterSubsForm.tsx” for taking user input as below in our app which we have used in previous blog post too.
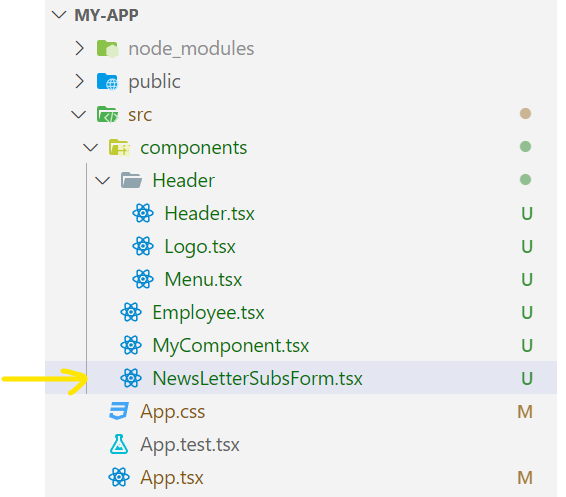
and here is some initial code which I have added to the “NewsLetterSubsForm.tsx” component.
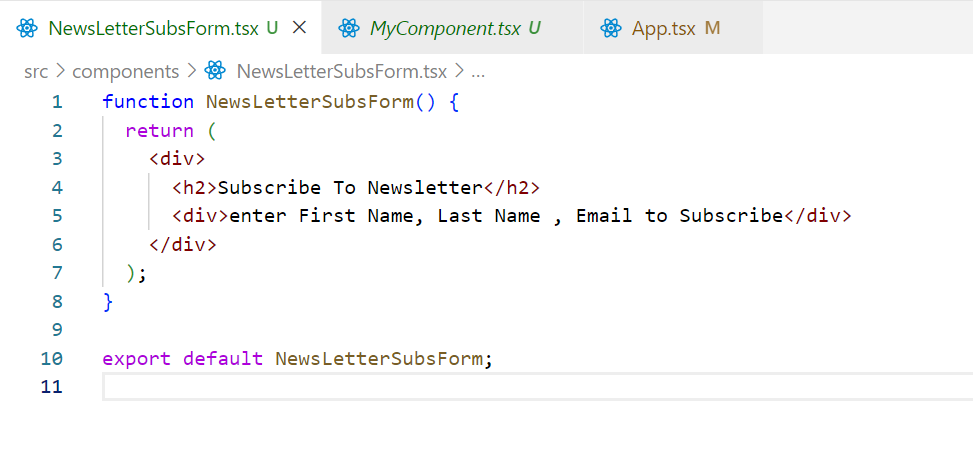
In the above code we have added a header information using h2 tag and the just added a div which is a placeholder to First Name, Last Name and Email input.
Lets update our App.tsx to show this component on the UI.
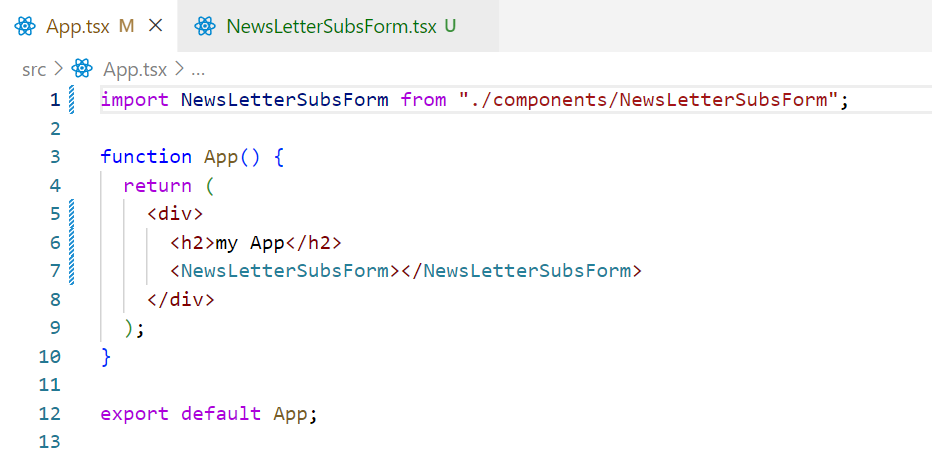
Lets run the application to see the output on browser.
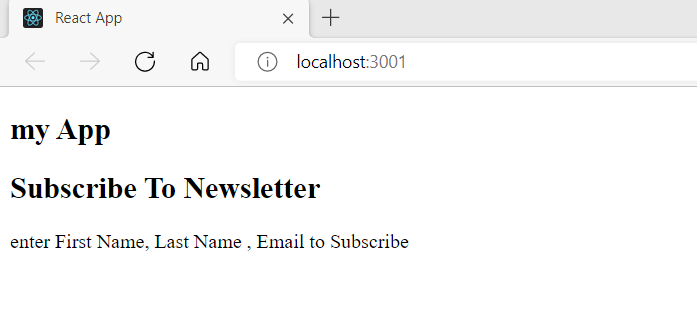
so, we are now able to render the component on UI. Lets start building the input form. Here is the updated NewsLetterSubsForm.tsx component code.
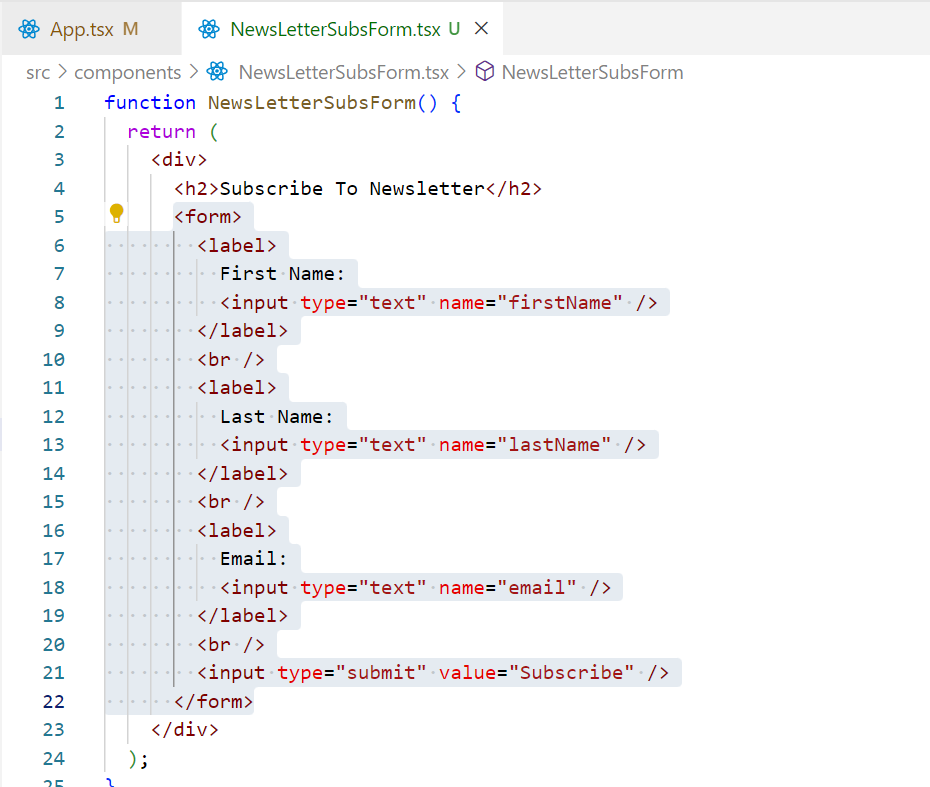
The highlighted code is the simple React form and it looks like as below on browser(lets ignore the CSS for now).
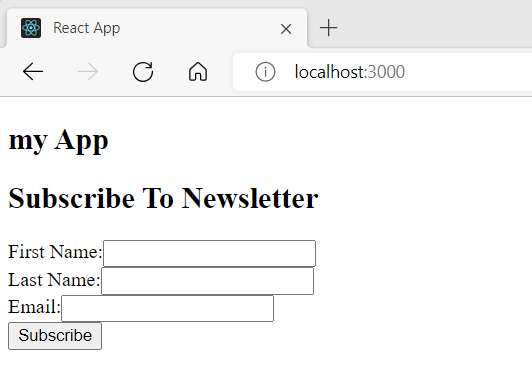
Now we need to add event handler to which will update the value when user is entering the values for First Name, Last Name or Email . Here is the updated code for NewsLetterSubsForm.tsx component
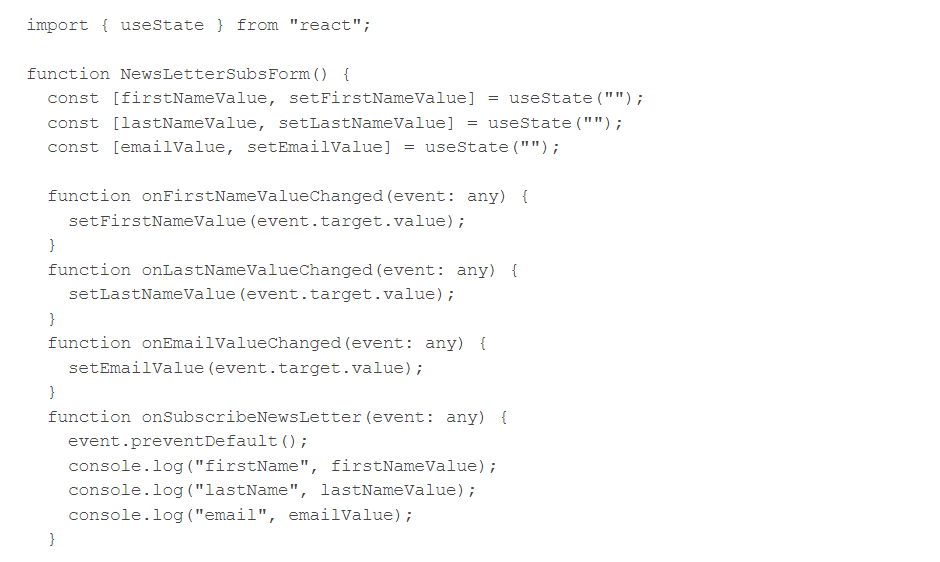
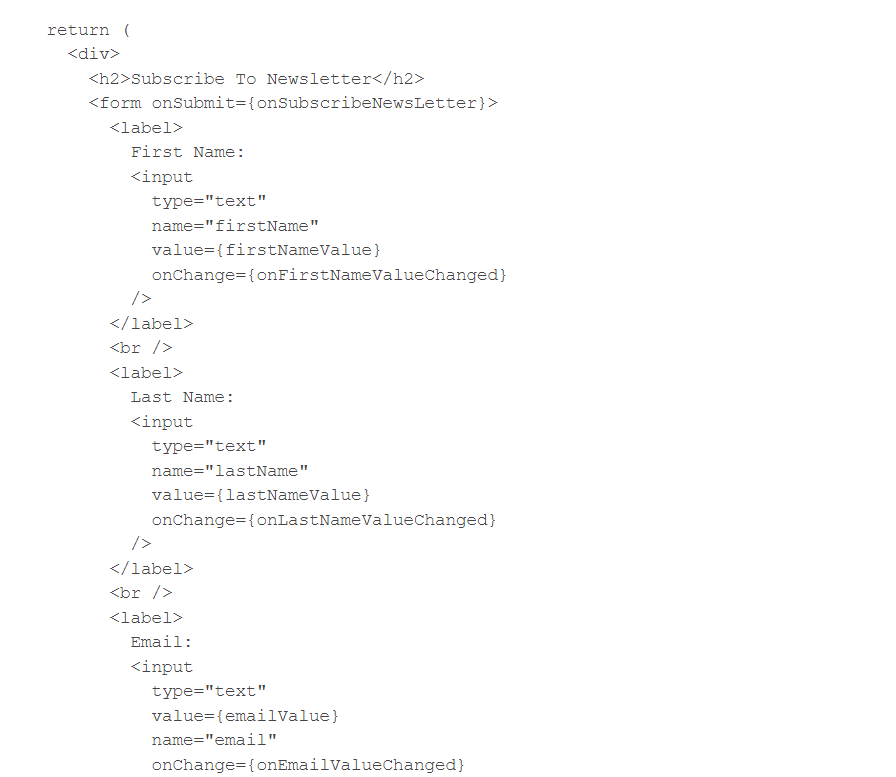
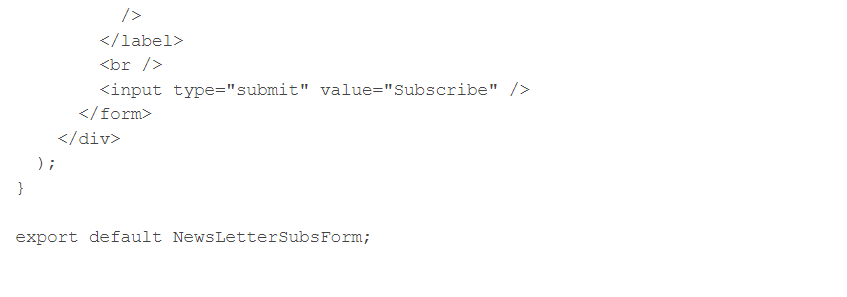
Lets try to understand what is happening in above code. We are importing the useState at first. then at start of the NewsLetterSubsForm function we are defining three separate state objects which will be managing each control, FirstName, LastName and Email values separately. so here one component having multiple state objects dealing with different control value. now, after that we have defined two different event handlers named as “onFirstNameValueChanged” , “onLastNameValueChanged” and “onEmailValueChanged” which we are calling on firstName input control onChange, lastName input control and email input control respectively.
now if you look the input element we also binded the value with the respective state variable. i.e. , firstName input element value property binded with firstNameValue , lastName input element value property binded with lastNameValue and email input element value property binded with emailValue.
why we are doing like that, what I mean, we are binding onChange handler for dealing with input element value change and then storing that in a state object, again we are binding the value property to show that same state object value. The answer is , this will show the updated value on UI and keep the state also updated with correct value. This is an example of two way binding too.
ok, we missed the form element onSubmit event. we have attached another event handler named “onSubscribeNewsLetter” to deal with the submit event which will be triggered when the “subscribe” submit button clicked. If you look the “onSubscribeNewsLetter” function code. you will notice the first line which is

We added this line as it is preventing the form default behavior and not reloading the form on submit. One more thing, each event handler having the current event object by default when that occurs and this is default by React.
with this explanation, lets run the code and try subscribe after filling the form.
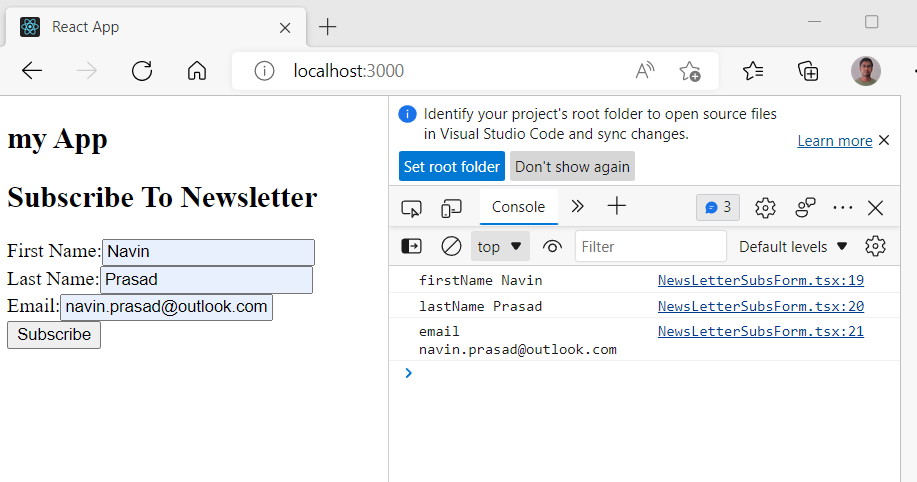
so you can see we are able to collect the different React input element values . This is one way to deal with multiple input element. The another way could be you can use one state object to keep all element value , that state object can keep the different element to keep track of different element values. Lets update the code for using one state object to deal with multiple element values as they are related ,i.e, all elements are collecting data related to user newsletter subscription.
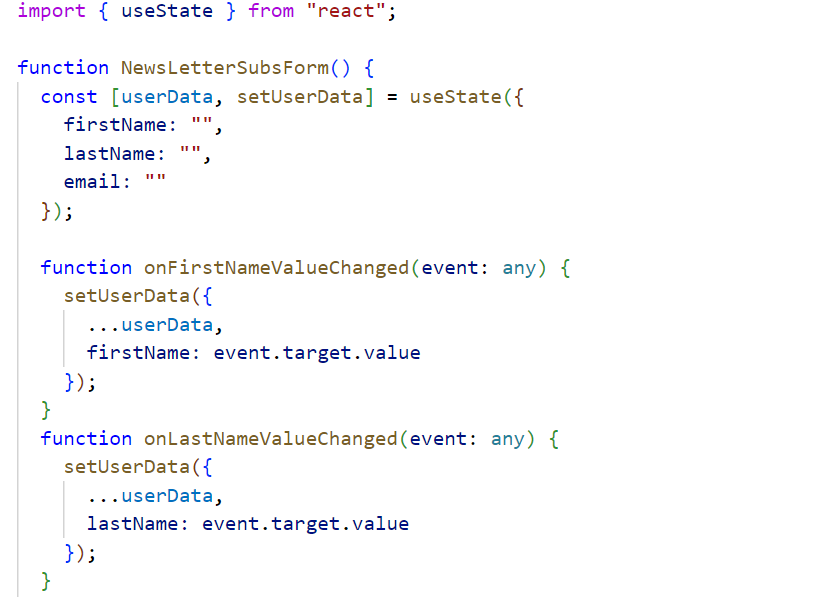
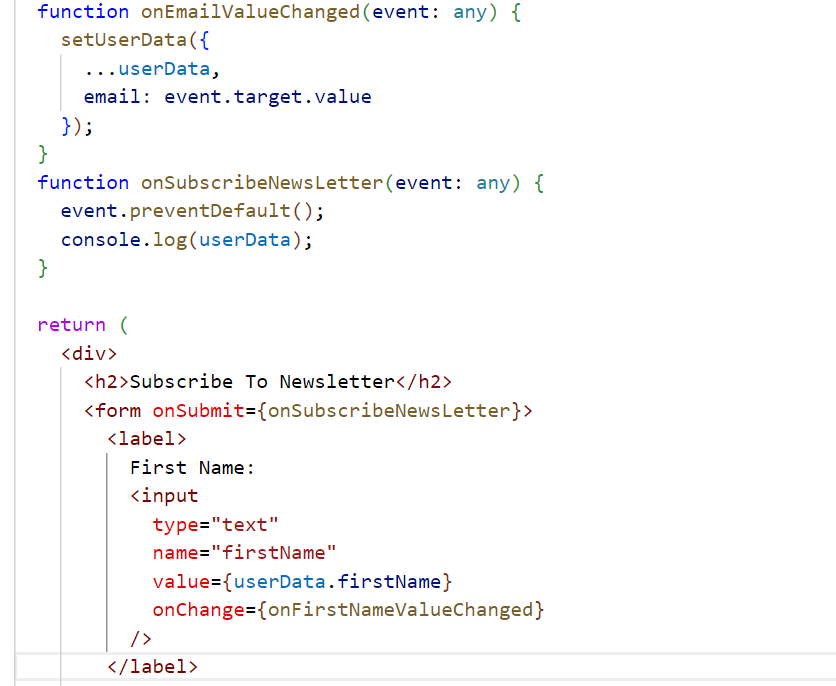
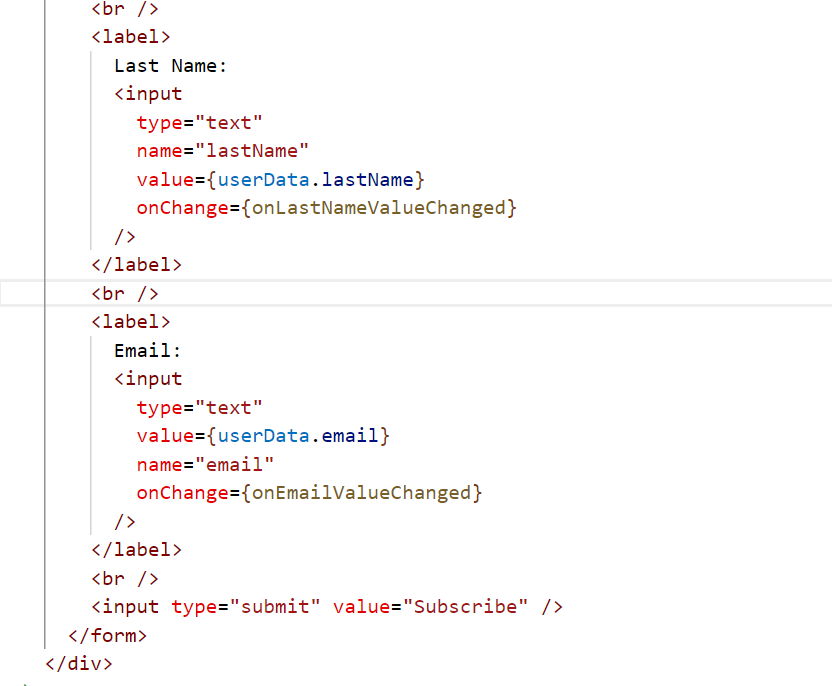
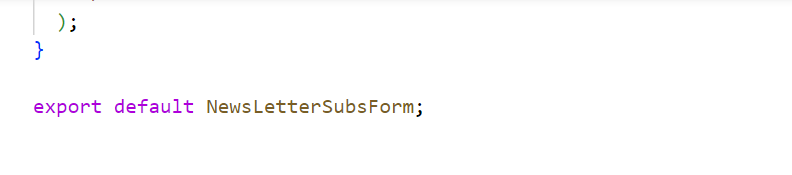
Lets try to understand above code. In the above code , we are using one State object to keep the updated value. We are also setting the initial values like { firstName: “”, lastName: “”, email: “” }
Next look the way to are using the setUserData method. This set method using the JavaScript spread operator to copy the values first and then we are overriding the desired field value in each control individual event handlers. for example , in onFirstNameValueChanged event handler which deals with firstName input element value change, we have code as below
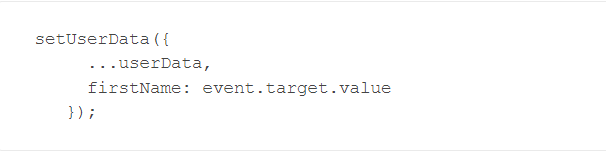
where we are calling the setUserData method first , then we are copying values using JavaScript spread operator like …userData and then we are updating the firstName field value in State like firstName: event.target.value
next, we also update the input element value property to take value from the userData object, for example on firstName input the code is like value={userData.firstName}
Lets see how it is handling the input on browser.
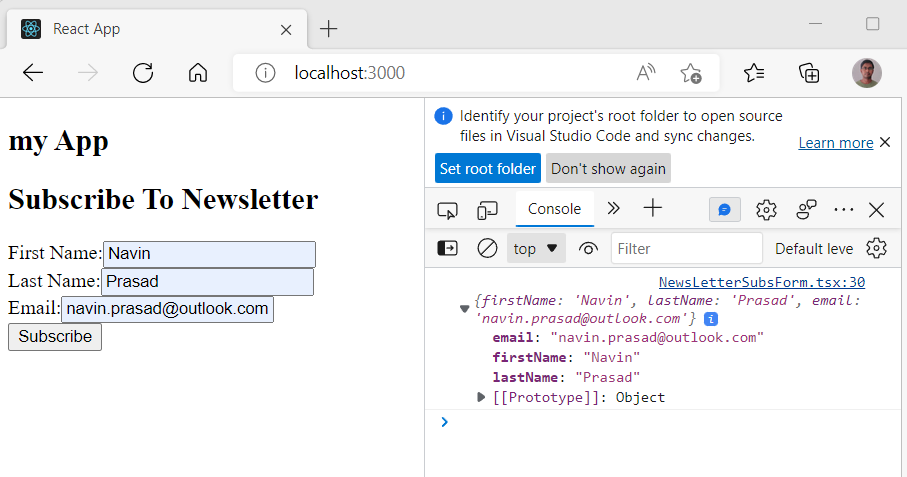
so, the form is working correctly. so , here we are using one component State to handle multiple input element values
There is one problem in above code , the JavaScript spread operator does not guarantee you that if every event handler will have current values, specially if your form is big and you are multiple elements to collect and update the values.
React provides a better way for dealing with such situation. by passing an update function on set method instead of object with spread operator . Let update the code with new and better way. Here is the updated code.
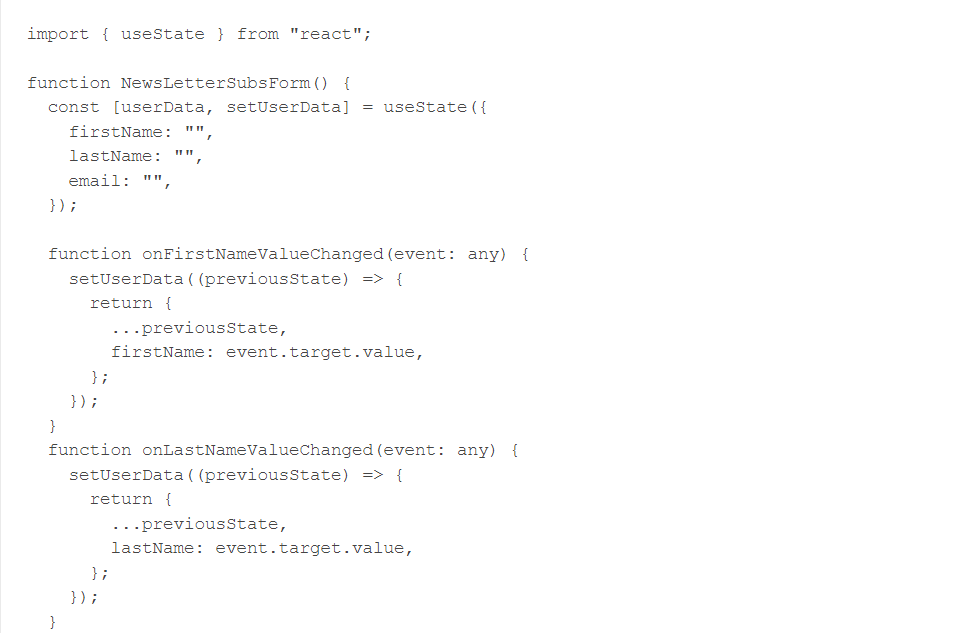
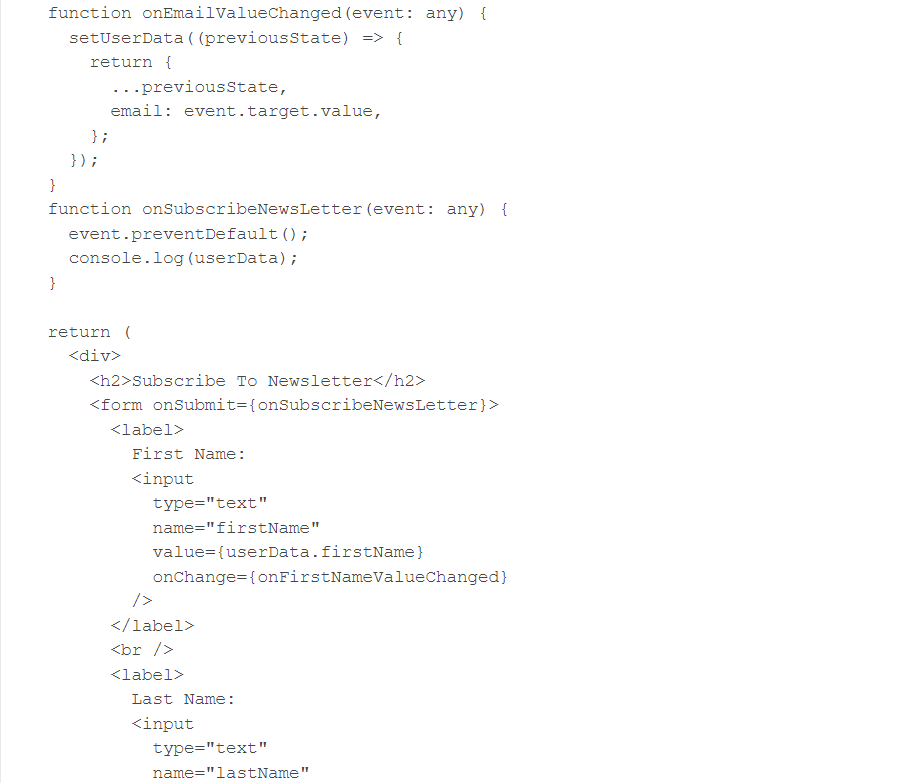
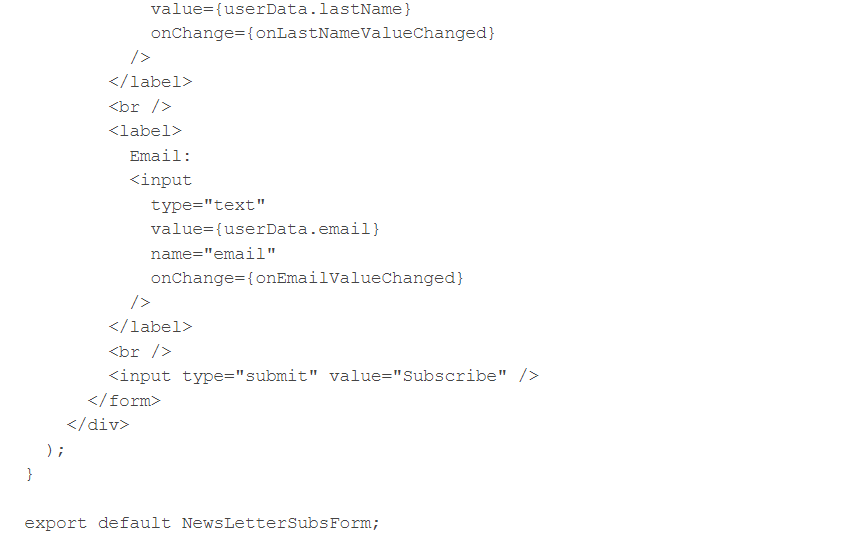
In the above code if you look how we are using setUserData method then you will notice that we are passing an anonymous function to setUserData instead of object. with this new syntax, React will guarantee to give the previousState which is the last updated state as parameter to that anonymous function and then are returning the new updated state using return statement and spread operator with previousState parameter. for example here is the code in onFirstNameValueChanged method .
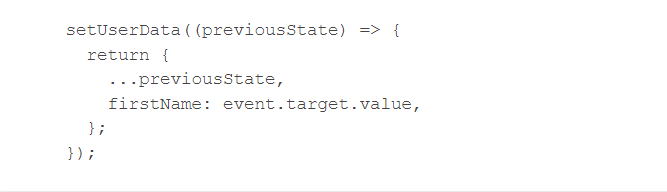
In the above code as explained we are getting the last updated state as parameter by React in previousState parameter and then in return we are returning the updated state and this new state is set by setUserData method to userData object.
Lets run the updated component code to see how it is working for user input.
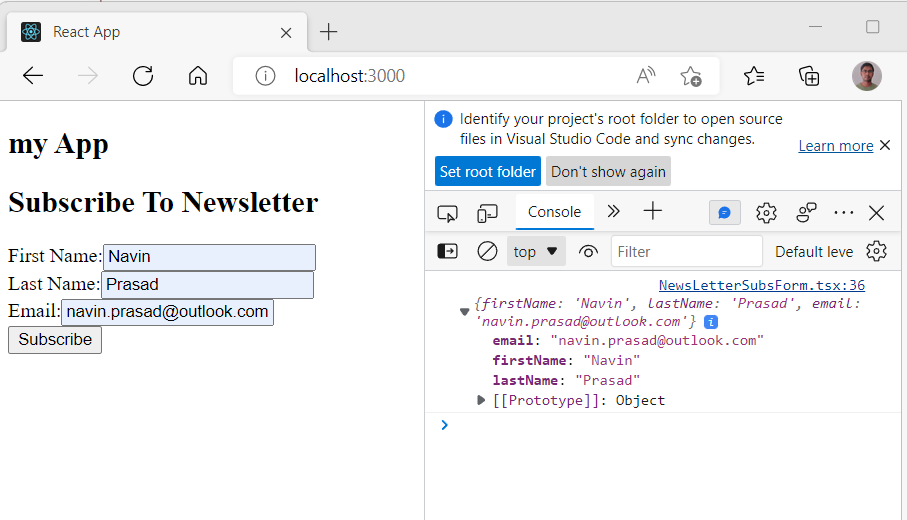
Nice, It is working correctly and we are getting same output as previous code.
So, We have learned working with multiple react form input and different ways of using component state in great detail.
Thanks and Happy Learning :). Please give your valuable feedback and share this post if you liked and learned something from this post.
2 thoughts on “Working With Multiple React Form inputs And Different Ways Of Using Component State In React- A Complete Guide”